· blog · 1 min read
How to show a Material-UI V4/V5 Tooltip at the cursor
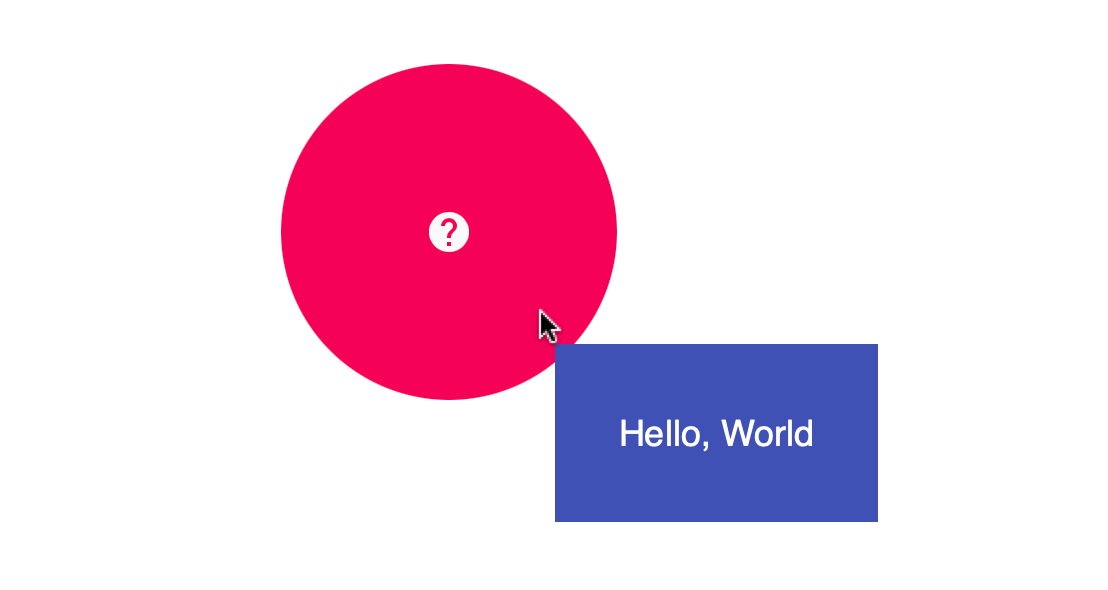
Recently, I needed to place a tooltip at the tip of the cursor, and it took a little bit of trial and error to get it to work. Here is the code I used.
Material-UI V4 Tooltip
Material-UI V4 uses the older V1 of the Popper.js library. To get it to work, I followed the mouse and put its position
in state, and then used the position to adjust the Tooltip’s Popper using the computeStyle
function:
const computeStyleFn = (data) => {
return {
...data,
styles: {
...data.styles,
left: `${position.clientX + 7}px`,
top: `${position.clientY + 2}px`,
},
};
};
See a rudimentary React 17 demo repo in action at CodeSandbox.io, or find the code on GitHub.
Material UI V5 Tooltip
With Material UI V5, the Popper.js library has been updated to V2. It has a followCursor
option available, so all we need to do here is figure out the offset that we want.
<Tooltip
followCursor={true}
PopperProps={{
modifiers: [
{
name: "offset",
options: {
offset: [88, 2],
},
},
],
}}
See a React 18 demo repo in action at CodeSandbox.io, or check out the code on GitHub.